On this page
In UNE for ArcGIS you can use attribute rules to improve data accuracy and speed up data capture by dynamically calculating data field values. You can also use rules to tailor the data collection and editing experience.
The following is a list of the different types of rules which can be applied to fields:
- Display Rules – Control whether and how a field is displayed to an end-user.
- Field Calculations – Automatically calculate a value for a field.
- Splitting and Merging – Control the resultant field value after a feature is split or merged.
- Validation – Configure custom validation checks and error messages to indicate whether a field value is valid.
- Advanced Attribute Rules – Configure additional logic to work with Domains, set default values, provide lookup lists, add field corrections, configure shared editing and auto balancing fields.
Adding an attribute rule
- A) From the Wireframe select the Properties panel.
B) This will open the Properties pane which will show all the layers in your web map. Click on the layer you want to configure an attribute rule for. - A) Now select the field you would like to add the rule to.
B) The rule pane will now show the options for rules you can apply to the selected attribute.
Some fields will have different available attribute rule options based on the data type (e.g. text vs numeric fields).
Display rules
Display rules allow you define how the field should be displayed to the user. To configure these display rules in the rule pane there is a section titled Display. Click on this to see the options.
- Hidden: The field will not be visible in the feature’s properties when it is hidden.
- Read-only: The field will not be editable in the feature’s properties.
- Multiline editor*: The field will expand to allow the value to go over multiple lines, showing a scrollbar if needed.
- Portal user*: As the user starts typing, the field will show a drop down list with portal users matching the typed search string. The user can then select one of them. Other values are not allowed.
- Display time**: The field will display the time after the date.
*only applies to text fields
**only applies to date fields
Conditional display rules
Hidden and Read-only rules can be set based on a condition determined using a script. When the script returns true, the field will be set to hidden or read-only mode. If the script returns false, the display rule will not apply.
For example the below script hides a field when another attribute of the feature is of a specific value.
// Hide this field, when the field developmentType is set as residential.
if( $feature.developmentType == "residential" ) {
// hide the field
return true
} else {
// the development type is not residential, therefore display the field.
return false
}
Calculation
Attribute calculation will calculate a field value automatically. If there are any changes to the components used in the calculation, it will run again to ensure that the data is correct. For example, if you use area in your calculation and the feature is reshaped, the field will automatically be recalculated. When a field has a calculation on it, an end user will not be able to change its value manually.
The following options are available:
- Unique ID when first created
- Determine using a script
Calculation script examples:
Simple Calculation
// Calculate the area of a feature in Hectares
return area(geometry($feature), "hectare");
Splitting and merging
When splitting or merging a geometry feature, you may want to automatically calculate attributes. To do this you can use the following:
- Calculate when splitting: This rule can be used to automatically calculate new values for child features, when the parent feature is split. This is useful if you wish to apportion field values between the new child features.
- Calculation when merging: This rule can be used to automatically calculate new values for a feature, when the features are merged.
Splitting and merging script examples:
Splitting Calculation
/** Apportion the field totalAmount by the area of this child compared
* to the original parent feature
*/
return (
($splitParent.totalAmount * Area(Geometry($feature))) /
Area(Geometry($splitParent))
);
Merging Calculation
/**Apportion each merged features contribution based on area.
*
* This method weights the relative contribution of each feature to the final
* total based on its individual area normalised by the final merged area.
*/
var total = 0;
// Get the full area of the merged feature
var totalarea = Area(Geometry($mergedFeature));
// Now go through all the features that were merged
// and use their area of the total, to apportion their contribution.
for (var f in $mergedFeatures) {
total += (Area(Geometry(f)) / totalarea) * f.IncomePotential;
}
return total;
Validation
Validation will allow you to define valid values for a field. If the user enters an incorrect value, then an error will be displayed to encourage the user to change it.
If the validation script returns true
, the attribute value is valid, if it returns false
then the value is not valid and an error message is returned.
Custom validation error message
To create a custom error message to the user, you can choose to return a string instead of false in the script as seen in the example below:
// Validate that a field attribute value is between 10 and 20.
// - If the value is not valid return a custom error message.
if( f < 10 || f > 20 ) {
// value is valid
return true
} else {
// value is not valid, so return a custom error message
return "Value " + f + " is not allowed."
}
Advanced attribute rules
In advanced rules you can choose the following attribute rules to define additional advanced behaviours.
Domain values
For a field which has an attribute domain, it is possible to define what values should be available from the domain list. The script can return a valid array of choices to limit the selection to, or can return null
to indicate that the full choice list should be returned.
/** Change the available choices from a domain list based on the value
* of another Field value.
*
* In this example if the field TestField is ORA, then restrict the domain
* to 3 choices. Otherwise return null (which means the full choice list
* available on the original domain).
*
* Arcade has in-built helper functions for working with domains:
* - Domain, DomainName, DomainCode
*
* @returns - The Arcade Script expects an array[] of choices to be returned.
*
*/
if($feature.TestField == "ORA"){
return ["EU", "EX", "EN"]
} else {
return null // return full domain choice list
}
Default values
Rather than define a default value in the data schema, you can dynamically assign a default value through a script. Any new feature created will have this value for the field by default.
// Set the default value to todays date
return Now();
This default value rule will take precedence over the value set in the feature template for a new feature.
Provide custom choice list or lookup
Configure a dynamic list of options for a user to select from on a field or add the capability for a user to search for a value in a lookup table.
Scripted choice
Provide a script which returns the valid choices and whether the list is static or may change. See the below script for an example:
/**Define what values should be available for an attribute.
*
* @return {choices[ {value: string, label:string}, {},.. ],static:boolean}
*/
return {
choices: [
{
value: "A",
label: "AAA"
},
{
value: "B",
label: "BBB"
},
{
value: "C",
label: "CCC"
}
],
static: true // If static, the script will only ever execute once.
//Use for lists that do not change
};
Scripted lookup
Provide a capability for a user to search for values based on values in a Lookup Table. The below image shows the result of a lookup rule configured to work against a hosted feature service containing country names.

/** Generate a scripted lookup for attribute values in a field.
*
* This example demonstrates creating a lookup table based on country names
* found in an externally hosted 'Countries Feature Service'.
*
*/
// Get the Countries Feature Service
var countries = FeatureSetByPortalItem(
"your item ID",
0,
["*"],
false
);
// If doing a reverse lookup
if ($lookuptype == "id") {
// Find the Country with the correct ISO Code
var idm = $lookupid;
var filt = Filter(countries, "ISO=@idm");
if (Count(filt) != 1) {
return null;
}
// Return its friendly text description
return First(filt).country;
} else if ($lookuptype == "search") {
// If doing a Search
// Prepare the SQL Query
var adm = Upper($searchtext) + "%";
// Get the top alphabetically ordered list of countries which match the search
// text
var filt = Top(
Orderby(Filter(countries, "UPPER(COUNTRY) LIKE @adm"), "COUNTRY ASC"),
100
);
// Loop through each element of the ordered list building a result set.
var ic = 0; //index to be incremeted
var result = []; //results list
for (var n in filt) {
result[ic] = { value: n.iso, label: n.country };
ic++;
}
return result;
}
Auto balance
If using a related table, auto balance will become available for a field to define a shared numeric attribute such as a percentage.
Shared editing
If you select multiple features, you can choose whether to edit shared properties at the same time. This is on by default.
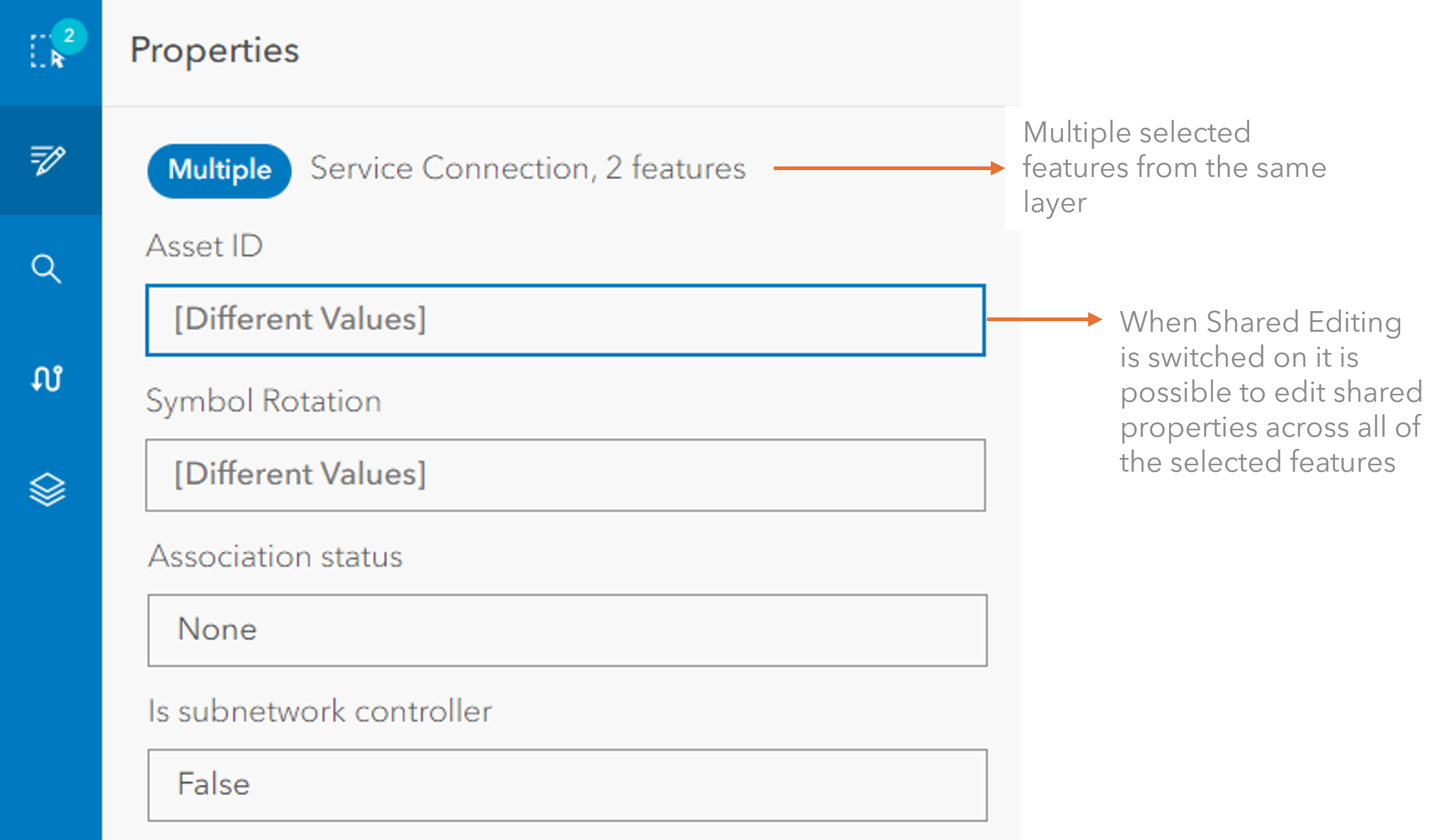
Field correction
It is possible to apply a field correction calculation for after a user has input data to a field. A simple example use case for this would be to correct the casing of input text as shown in the example below:
// Ensure that an input name field capitalises only the first letter.
var inputFieldValue = $feature.FirstName
// Value with field correction applied
var correctedValue = ""
for( var i = 0; i < Count(inputFieldValue); i++ ){
if( i == 0){
//convert first letter to upper case
correctedValue += Upper(inputFieldValue[0])
}
else {
//convert subsequent letters to lower case
correctedValue += Lower(inputFieldValue[i])
}
}
// example: joNaThan becomes Jonathan.
return correctedValue